Solana: How to overcome the 1000 transaction limit using getSignaturesForAddress?
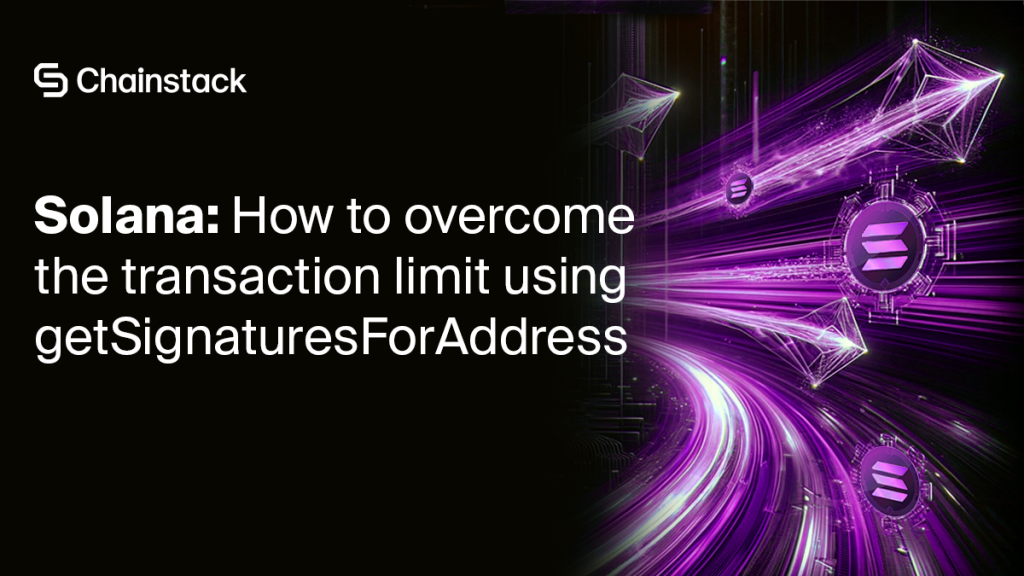
Handling large transaction lists can be a daunting task. This is especially true for Solana, as the protocol is only able to fetch up to 1000 transactions at a time. Because of this, fetching the history of transactions for a given wallet address becomes rather problematic.
But don’t worry, there’s a way to overcome this limitation using JavaScript and the Solana Web3.js library. This guide will provide you with an in-depth look at how to handle large transaction lists in Solana using the getSignaturesForAddress
function. Let’s get started!
What is the getSignaturesForAddress
method?
The getSignaturesForAddress
method is a part of the Solana Web3.js library, a JavaScript library that allows you to interact with the Solana blockchain. This method retrieves an array of transaction signatures for transactions involving the specified account. The transactions are sorted by block height in descending order, providing a chronological view of the account’s transaction history.
Here’s a basic example of how to use getSignaturesForAddress
:
- Sign up with Chainstack.
- Deploy a node.
- View node access and credentials.
- Process dependencies and create the function:
const solanaweb3 = require('@solana/web3.js');
const connection = new solanaweb3.Connection(solanaweb3.clusterApiUrl('mainnet-beta'));
async function fetchTransactions(address) {
const pubkey = new solanaweb3.PublicKey(address);
const signatures = await connection.getSignaturesForAddress(pubkey);
console.log(signatures);
}
fetchTransactions('YourWalletAddressHere');
In this example, you first import the Solana Web3.js library and establish a connection to the Solana blockchain. You then define an asynchronous function fetchTransactions
that takes a wallet address as an argument. Inside this function, you convert the address into a public key and use getSignaturesForAddress
to fetch the transaction signatures. Finally, you log the signatures to the console.
getSignaturesForAddress
pagination how to
The getSignaturesForAddress
method in Solana’s Web3.js library comes with a built-in limitation: it can only fetch up to 1000 transactions per request. This is where pagination comes into play. Pagination is a technique that allows you to fetch data in smaller, manageable chunks, rather than retrieving all the data at once.
In the context of Solana, pagination is supported via the before
and until
parameters in the getSignaturesForAddress
method. These parameters allow you to specify a range of signatures, effectively controlling the number of transactions fetched in each request.
For instance, if you want to fetch transactions that occurred before a certain transaction, you can use before
and provide the signature of the transaction as its value. Similarly, until
allows you to fetch transactions that occurred up until a certain transaction.
By using these options, you can fetch more than 1000 transactions by making multiple requests, each fetching a different range of transactions. This is a crucial technique for handling large transaction lists in Solana.
How to use a loop to automate data retrieval
To automate the process of fetching all transactions for addresses with extensive histories, you can use a recursive function or a loop. This approach continuously fetches transactions until all data has been retrieved. Here’s a step-by-step breakdown of how to implement this using a loop:
- Initial fetch: Start by fetching the first 1000 transactions using
getSignaturesForAddress
. - Review and continue: After each fetch, check if the number of transactions is less than 1000. If it is, you’ve reached the end of the transaction history. If not, you need to continue fetching.
- Recursive fetch: Use the signature of the last transaction from the fetched list as the
before
parameter in the nextgetSignaturesForAddress
request. This will fetch the next batch of transactions. Repeat this step until all transactions are retrieved.
Here’s a complete code example that implements this approach:
const solanaweb3 = require('@solana/web3.js');
const connection = new solanaweb3.Connection(solanaweb3.clusterApiUrl('mainnet-beta'));
async function getAllTransactions(address) {
const pubkey = new solanaweb3.PublicKey(address);
let allTransactions = [];
let fetchedTransactions;
let lastSignature = null;
do {
fetchedTransactions = await connection.getSignaturesForAddress(pubkey, {
before: lastSignature,
limit: 1000
});
allTransactions.push(...fetchedTransactions);
lastSignature = fetchedTransactions.length > 0 ? fetchedTransactions[fetchedTransactions.length - 1].signature : null;
} while (fetchedTransactions.length === 1000);
return allTransactions;
}
getAllTransactions('YourWalletAddressHere').then(transactions => {
console.log('Total transactions fetched:', transactions.length);
});
In this example, you define an asynchronous function getAllTransactions
that fetches all transactions for a given address. Inside the function, you use a do-while loop to continuously fetch transactions until all have been retrieved.
After each fetch, you check if the number of fetched transactions is less than 1000. If it is, you’ve reached the end of the transaction history. If not, you use the signature of the last transaction as before
in the next getSignaturesForAddress
request.
This ensures that you fetch the next batch of transactions in the next request. Finally, you log the total number of fetched transactions to the console.
Large transaction list handling best practices
Retrieving a large number of transactions can be resource-intensive and time-consuming. Therefore, it’s important to consider performance when implementing a solution for handling large transaction lists in Solana. Here are some tips to handle large data efficiently:
- Rate limiting: Solana’s public and provider RPC servers impose rate limits to prevent abuse (find ours here). If you’re making a large number of requests in a short period of time, you might hit these limits. To avoid this, consider using a Chainstack node or adding delays between requests to spread out the load.
- Concurrency: If you’re processing transactions in parallel, it’s important to implement concurrency control. This can prevent your system and your RPC node from being overloaded with too many simultaneous requests. You can do this by limiting the number of concurrent requests or using a queue to manage requests.
- Logging: Adding logging at critical steps can help you monitor the progress of your transaction fetching process. It can also help you troubleshoot any issues that might arise during the process. For instance, you can log the number of fetched transactions after each request, the total number of transactions fetched so far, and any errors that occur during the process.
By considering these performance aspects, you can ensure that your solution for handling large transaction lists in Solana is not only effective but also efficient and reliable.
Further reading
Expand your Solana knowledge and skills with these comprehensive Chainstack resources:
- Troubleshooting common Solana errors master guide: Learn how to tackle common Solana errors like Rust version conflicts, Borsh serialization issues, blockstore errors, and more.
- The ultimate Solana developer guide: Master Solana development all across the board with this ultimate guide, covering everything from pastable snippets to advanced integrations.
- Mastering Solana series: Explore Solana essentials like token swaps with Raydium SDK, SPL token transfers, account retrieval methods, and getTokenLargestAccounts RPC insights.
- Solana-web3.js Tutorial: Learn how to use the solana-web3.js method in just 7 minutes to mint an SPL token, transfer tokens, and delegate tokens.
- Querying and analyzing Solana data from Raydium with Python: Learn about Raydium, a leading DEX on Solana, and explore how DeFi enables token exchanges across chains and fiat.
- Powerful Chainstack infrastructure optimized for Solana: Run high-performing Solana RPC nodes and APIs in minutes on a platform built for scale with Chainstack.
- How to run a Solana RPC node: Learn how to run your Solana RPC node instance on Chainstack, connected to the mainnet beta and complete with metric monitoring.
- Solana tool suite: Learn how to interact with your Chainstack Solana node and develop DApps.
- Solana glossary: Get a better understanding of key Solana terminology and its definitions.
Bringing it all together
Handling large transaction lists in Solana can be a challenging task due to the 1000 transaction limit of the getSignaturesForAddress
function. However, with the right approach, you can overcome this limitation and fetch all transactions for a given address. By using a loop to paginate through results from getSignaturesForAddress
, you can effectively manage and retrieve extensive transaction histories on the Solana blockchain.
This approach is invaluable for developers working on applications that require access to complete transaction data. It provides a robust foundation for building reliable and efficient Solana programs. Remember, while this guide focuses on JavaScript and the Solana Web3.js library, the principles and techniques discussed can be applied to other languages and chains as well.
So, whether you’re developing a high-frequency trading platform or a popular Solana DApp, don’t let large transaction lists slow you down. With the right tools and techniques, you can handle any amount of transaction data with ease. Happy coding!
Power-boost your project on Chainstack
- Discover how you can save thousands in infra costs every month with our unbeatable pricing on the most complete Web3 development platform.
- Input your workload and see how affordable Chainstack is compared to other RPC providers.
- Connect to Ethereum, Solana, BNB Smart Chain, Polygon, Arbitrum, Base, Optimism, Avalanche, TON, Ronin, zkSync Era, Starknet, Scroll, Aptos, Fantom, Cronos, Gnosis Chain, Klaytn, Moonbeam, Celo, Aurora, Oasis Sapphire, Polygon zkEVM, Bitcoin and Harmony mainnet or testnets through an interface designed to help you get the job done.
- To learn more about Chainstack, visit our Developer Portal or join our Discord server and Telegram group.
- Are you in need of testnet tokens? Request some from our faucets. Multi-chain faucet, Sepolia faucet, Holesky faucet, BNB faucet, zkSync faucet, Scroll faucet.
Have you already explored what you can achieve with Chainstack? Get started for free today.