Solana-web3.js Tutorial – Learn In Just 7 minutes
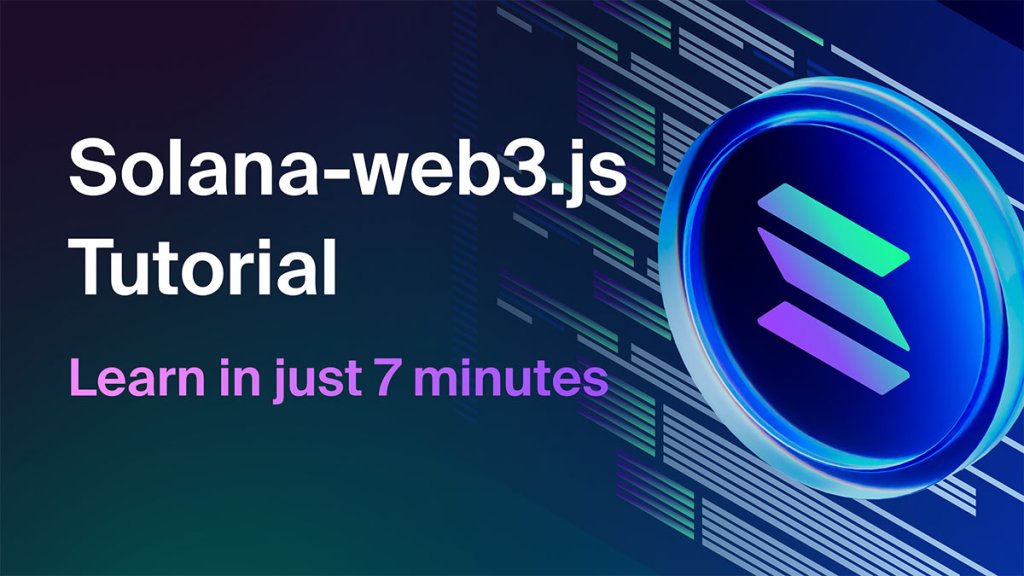
In this tutorial, we’ll go through some examples of basic utilization of solana-web3.js. This tutorial is derived from a previous episode of Byte-Size BUIDLing.
Solana-web3js is among the most popular methods of connecting to the Solana blockchain and building applications. Because of this, it’s really important to know what the library is capable of as well as how to actually use it.
In an effort to make this topic a little bit easier to digest, we will go through three key examples of solana-web3.js utilization.
What we will cover in this tutorial
1. Minting an SPL token
2. Sending a normal SOL transaction
3. Delegate SOL natively through solana-web3.js.
We will be covering a lot of ground here, so we will go through things very quickly, but if you’d like to dive more into the specific concepts, we do already have tutorials on both minting SPL tokens & sending a transaction with solana-web3.js.
So, let’s jump right into it.
Import key libraries to get started
const solanaWeb3 = require('@solana/web3.js');
const splToken = require('@solana/spl-token');
consta bs58 = require('bs58');
Ok, so now we’ve imported solana-web3.js, spl-token, and base58.
We’ll be using solana-web3 for most of this, as a base layer connection and interactivity layer with the Solana blockchain.
We’ll be using spl-token for the minting and tokenAccount process, and we’ll be using base58 for the private key decoding.
Write the code for our main asynchronous function.
async function main() {}
Define connection variable
This will be the variable that we will use our RPC endpoint to connect to the Solana blockchain itself.
If you do not have access to a node, you can deploy a high-performance node for free using Chainstack.
After you sign up, you will need to create a project, join a network, and then you will have access to your own HTTPS and WSS endpoints. Here’s a tutorial that we’ve made for Scroll network, but the steps are the same for any protocol that we support. Just select Solana in the “Join network” stage instead of Scroll. https://twitter.com/ChainstackHQ/status/1693653770859659613?s=20
const connection = solanaWeb3.Connection("https://nd-729-020-604.p2pify.com/89254f3e5bf61205d9558141245b8a80", {wsEndpoint:"wss://ws-nd-729-020-604.p2pify.com/89254f3e5bf61205d9558141245b8a80"});
Define the main account
const walletKeyPair = solanaWeb3.Keypair.fromSecretKey(new Uint8Array(bs58.decode(process.env.PRIVATE_KEY)));
Now we have our main wallet, let’s go and quickly run a balance call on it, just so we know how much SOL we’re working with.
let balance = await connection.getBalance(walletKeypair.publicKey);
console.log(balance / solanaWeb3.LAMPORTS_PER_SOL);
Before we continue we can run this code and make sure that it works. After calling the main() function you should see how much SOL you have in your wallet. We have about 0.3 SOL in our wallet right now.
main()
solana-web3.js utilization – minting an SPL token
Defining a mint variable.
const mint = await splToken.createMint(
connection,
walletKeyPair,
walletKeyPair.publicKey,
null, // this is the freeze authority
9, //decimals
undefined,
{},
splToken.TOKEN_PROGRAM_ID,
);
Create a token account
const tokenAccount = await splToken.getOrCreateAssociatedTokenAccount(
connection,
walletKeyPair,
mint,
walletKeyPair.publicKey,
);
Mint the token itself
await splToken.mintTo(
connection,
walletKeyPair,
mint,
tokenAccount.address,
walletKeyPair.publicKey,
1000000000000,
)
Alright, so, we’ve created our mint object which runs the createMint() method on the SPL token library that we imported, then we created a tokenAccount, and we minted the tokens to our wallet.
Before we continue, let’s run the code and make sure that it works by calling the main() function again.
You should receive 1000 tokens of the SPL token that we’ve just created or a different amount if you input a different value in the mint function.
Sending a SOL transaction from one address to another using solana-web3.js
Generate a second address to send SOL to
Let’s generate a 2nd wallet
const secondWalletKeyPair = solanaWeb3.Keypair.generate();
Define a transaction object
const transaction = new solanaWeb3.Transaction().add(
solanaWeb3.SystemProgram.transfer({
fromPubkey: walletKeyPair.publicKey,
toPubkey: secondWalletKeyPair.publicKey,
lamports: solanaWeb3.LAMPORTS_PER_SOL * 0.001,
}),
);
Send the transaction and save the signature
const signature = await solanaWeb3.sendAndConfirmTransaction(
connection,
transaction,
[walletKeyPair],
);
Sweet, at this point, if you run the script it should create a new SPL token, mint 1000 of these tokens to your wallet, and it will also send 0.001 SOL to a fresh wallet that you generate in secondWalletKeyPair.
Feel free to give it a try and run the code by calling the main() function.
Delegate SOL natively through solana-web3.js.
This will be a little more complex than what we’ve done so far. In order to do it right, we will need 4 key components.
- Create a stake account.
- Initialize the transaction for the stake account creation.
- Delegate our stake.
- Initialize the transaction for delegation.
Create a stake account
const stakeAccount = solanaWeb3.Keypair.generate();
let createStakeAccountInstruction = solanaWeb3.StakeProgram.createAccount({
fromPubkey: walletKeyPair.publicKey,
stakePubkey: stakeAccount.publicKey,
authorized: new solanaWeb3.Authorized(walletKeyPair.publicKey, walletKeyPair.publicKey),
lamports: solanaWeb3.LAMPORTS_PER_SOL * 0.02,
});
Initialize the transaction for the stake account creation
let createStakeAccountTransaction = new solanaWeb3.Transaciton().add(createStakeAccountInstruction);
createStakeAccountTransaction.recentBlockhash = (await connection.getRecentBlockhash()).blockhash;
createStakeAccountTransaction.feePayer = walletKeyPair.publicKey;
createStakeAccountTransaction.partialSign(stakeAccount);
createStakeAccountTransaction = await solanaWeb3.sendAndConfirmTransaction(
connection,
createStakeAccountTransaction,
[walletKeyPair, stakeAccount],
);
Build and send the delegation transaction
We’ll first need to define the public key of the validator that we’re delegating to.
const votePubkey = new solanaWeb3.PublicKey('{input the public key here and remove curly brackets}')
let delegateInstruction = solanaWeb3.StakeProgram.delegate({
stakePubkey: stakeAccount.publicKey,
authorizedPubkey: walletKeyPair.publicKey,
votePubkey,
});
Initialize the transaction for delegation
let delegateTransaction = new solanaWeb3.Transaction().add(delegateInstruction);
delegateTransaction.recentBlockhash = (await connection.getRecentBlockhash()).blockhash;
delegateTransaction.feePayer = walletKeyPair.publicKey;
delegateTransaction.sign(walletKeyPair);
delegateTransaction = await solanaWeb3.sendAndConfirmTransaction(
connection,
delegateTransaction,
[walletKeyPair],
);
Recap of what you did
- Imported the base libraries.
- Established a connection to the Solana blockchain through a Chainstack RPC endpoint.
- Imported a wallet.
- Read the balance of that wallet.
- Minted an SPL token.
- Sent a SOL transaction.
- Staked 0.02 SOL.
Now, you can bring it all together and give it a try by calling main().
If you’ve got until this point, congratulations, you are on your way to becoming a better Solana developer.
If you’d like to take a deeper dive into the core concepts covered within this tutorial, you can find all three tasks here (minting SPL tokens, sending a transaction, and delegating SOL) covered in-depth on our developer portal.
- Minting SPL tokens with solana-web3.js
- Send Solana transaction using solana-web3.js
- Delegating SOL with solana-web3.js
Further reading
Expand your Solana knowledge and skills with these comprehensive Chainstack resources:
- The ultimate Solana developer guide: Master Solana development all across the board with this ultimate guide, covering everything from pastable snippets to advanced integrations.
- Mastering Solana series: Explore Solana essentials like token swaps with Raydium SDK, SPL token transfers, account retrieval methods, and getTokenLargestAccounts RPC insights.
- Troubleshooting common Solana errors master guide: Learn how to tackle common Solana errors like Rust version conflicts, Borsh serialization issues, blockstore errors, and more.
- Querying and analyzing Solana data from Raydium with Python: Learn about Raydium, a leading DEX on Solana, and explore how DeFi enables token exchanges across chains and fiat.
- Powerful Chainstack infrastructure optimized for Solana: Run high-performing Solana RPC nodes and APIs in minutes on a platform built for scale with Chainstack.
- How to run a Solana RPC node: Learn how to run your Solana RPC node instance on Chainstack, connected to the mainnet beta and complete with metric monitoring.
- Solana tool suite: Learn how to interact with your Chainstack Solana node and develop DApps.
- Solana glossary: Get a better understanding of key Solana terminology and its definitions.
Power-boost your project on Chainstack
- Discover how you can save thousands in infra costs every month with our unbeatable pricing on the most complete Web3 development platform.
- Input your workload and see how affordable Chainstack is compared to other RPC providers.
- Connect to Ethereum, Solana, BNB Smart Chain, Polygon, Arbitrum, Base, Optimism, Avalanche, TON, Ronin, zkSync Era, Starknet, Scroll, Aptos, Fantom, Cronos, Gnosis Chain, Klaytn, Moonbeam, Celo, Aurora, Oasis Sapphire, Polygon zkEVM, Bitcoin, Tezos and Harmony mainnet or testnets through an interface designed to help you get the job done.
- To learn more about Chainstack, visit our Developer Portal or join our Discord server and Telegram group.
- Are you in need of testnet tokens? Request some from our faucets. Multi-chain faucet, Sepolia faucet, Holesky faucet, BNB faucet, zkSync faucet, Scroll faucet.
Have you already explored what you can achieve with Chainstack? Get started for free today.