Solana: Master guide to troubleshooting common development errors
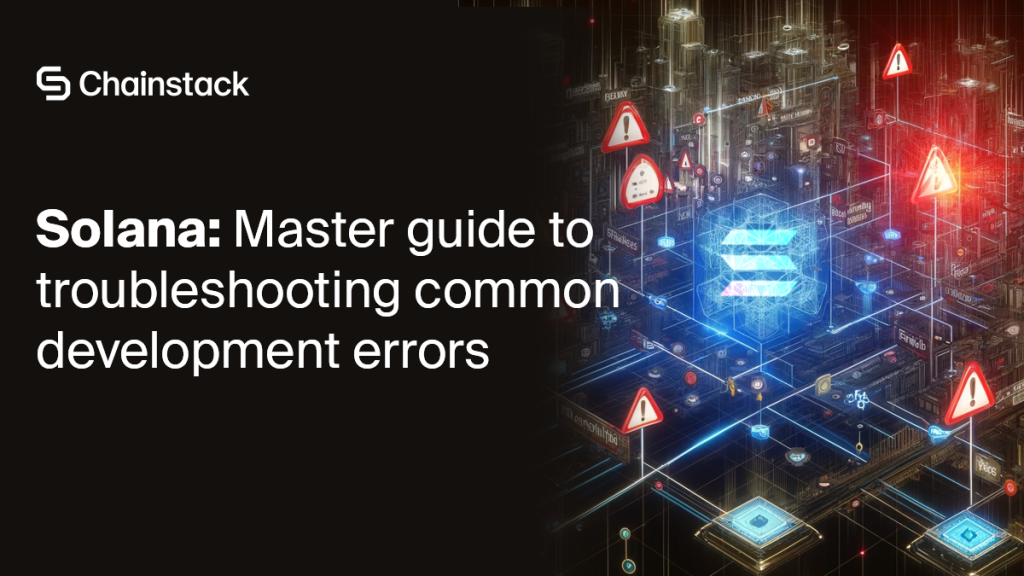
Solana is a powerful blockchain ecosystem that enables developers to build high-performance DApps. However, like any development platform, it comes with its challenges.
This master guide will help you troubleshoot common errors encountered during Solana development, including Rust version conflicts, Borsh serialization issues, blockstore errors, and module parse errors. By understanding these problems and following our step-by-step solutions, you can ensure a smoother development experience and focus on creating robust applications.
Let’s get started!
What are Rust version conflicts in Solana?
When working with Solana development tools, like Anchor, you might occasionally encounter versions clashing between your locally installed Rust compiler (rustc
) and the versions demanded by your Solana dependencies. The error messages can be cryptic, and the solutions not always straightforward.
The conflict arises mainly due to a mismatch between the rustc
version that a Solana package expects and the version that is currently running on your system. It’s perplexing, indeed, when your local rustc
version is newer than the one required and still, errors pop up.
Consider this scenario—you received an error message that reads:
*error: package solana-program v1.16.3 cannot be built because it requires rustc 1.68.0 or newer, while the currently active rustc version is 1.62.0-dev.*
To add to the confusion, your local Rust version shows as rustc 1.75.0
. This clearly indicates that the environment in which anchor
is running might not be using the global rustc
version.
Until now, this might’ve seemed like a perplexing puzzle to you, but in the following sections, we will help you piece this mystery together one step at a time.
What causes Rust version conflicts in Solana?
Version conflicts in Solana often trace back to a few common causes. Identifying these can be a critical first step on the path to resolution.
The first culprit often lies in having multiple Rust versions. You might install both a stable and a nightly or development version of Rust which can lead to conflicts. Issues can also arise when the system prioritizes one version of rustc
over another in the PATH environment variable, causing an Environment Path Priorities issue.
Moreover, sometimes the Rust version conflict roots back to your project configuration itself. The Toolchain Configuration in cargo.toml
might specify a different Rust version through a rust-toolchain
file or similar settings.
By pinpointing the source of the conflict, you can tackle the issue head-on. But diagnosis is just the first part. In the following sections, we will guide you step-by-step through the resolution process.
How to resolve Rust version conflicts in Solana?
Once you’ve identified the possible causes, it’s time to take action. Let’s tackle each issue, one step at a time.
Step 1: Verify the Rust version
Start by confirming the version of Rust installed globally. Execute the command rustc --version
to check the active Rust version. If it matches the latest version you believe you installed, move on to the next step.
Step 2: Check for multiple Rust installations
Detect additional Rust installations that might cause conflict by using the command which -a rustc
. This command lists all occurrences of rustc
in the order they appear in your PATH. A list with more than one version indicates potential trouble.
Step 3: Adjust the PATH environment variable
With multiple Rust installations, it’s crucial to ensure the path to the desired Rust version appears first in your PATH environment variable. Consider modifying your .bashrc
, .zshrc
, or equivalent shell configuration file to adjust the PATH order. The command export PATH="/path/to/desired/rust/bin:$PATH"
helps achieve this.
Step 4: Specify your Rust version in cargo.toml
Your project depends on a specific Rust version? Mention that in the cargo.toml
file using a rust-toolchain
file:
[toolchain]
channel = "1.68.0"
This step ensures Cargo uses the correct Rust version for your project.
Step 5: Use the rustup
override if needed
For project-specific Rust versions, apply rustup
to set an override. The command rustup override set 1.68.0
designates the Rust compiler version for the current project directory only.
Alternative solution: Downgrading the Solana package
In some situations, despite your efforts, version conflicts might continue to occur. If you find yourself unable to resolve the Rust version conflict, consider downgrading your Solana package to mitigate the issue.
As a last resort, to bypass the conflict, adapt your Solana tool suite to a version that aligns with your Rust environment. You can do this using the command solana-install init 1.16.3
. This command installs the specified version of the Solana tool suite.
Though it might seem like a step back, aligning your tools can often lead to a smoother development experience. Remember, the key to a successful development environment is harmony among the tools.
What are Borsh serialization errors in Solana?
Let’s get down to basics, shall we? When you encounter the message error[E0277]: the trait bound Pubkey: BorshSerialize is not satisfied
, it’s the Rust compiler waving a red flag at you. But, what for? It’s because it simply cannot find an implementation of the BorshSerialize
trait for the Pubkey
type within your project’s serialization framework.
Let’s break that down a bit. You see, in Solana programs, the Pubkey
type is frequently used to represent public keys of accounts. Seeing this error indicates that the implementation of the BorshSerialize
trait for the Pubkey
type is missing in your project, or it’s not properly visible.
But fret not, understanding this error is the first step towards solving it. Let’s move on to the next section, where we decipher what causes this error.
What causes Borsh serialization errors in Solana?
Troubleshooting an error often involves a journey of discovery back to its roots. Similarly, understanding the situations that commonly birth this error can save you a lot of time and headaches. Typically, the the trait BorshSerialize is not implemented for Pubkey
error emerges from one of these scenarios:
- Mismatched Borsh versions: It’s a chaotic world out there with mutable versions of Borsh library in the various crates of your
cargo.toml
. This version variance can bring about incompatibilities, giving rise to our error in question. - Incorrect or missing Borsh traits: In certain instances, your project configuration might have the required Borsh traits absent or incorrectly implemented. These traits might also go amiss due to updates in dependent crates.
Now that we have the potential culprits identified, let’s proceed further to diagnose these causes in detail. Ready to turn that error into a solution? On we move!
How to diagnose Borsh serialization errors in Solana?
Diagnosing this error is similar to a detective following clues to solve a mysterious case. The first clue is to confirm that all dependencies are correctly mentioned and compatible.
You can start this investigation by inspecting your Cargo.toml
and Cargo.lock
files. These files contain information about the versions of borsh
utilized by your dependencies.
For example, if a crate demands [email protected]
while another requires [email protected]
, you must ensure these versions do not compete or result in unsatisfied traits. By verifying the compatibility of Borsh versions, you can pinpoint where the issue originates.
Sharpen your detective skills and follow these clues. With the right diagnosis, you’re one step closer to resolving this error. The solution is almost within your grasp!
How to solve Borsh serialization errors in Solana?
As promised, here is a systematic approach to remedying the Borsh serialization error. Hold on tight, we’re off to a troubleshooting adventure!
- Verify project dependencies: Start at your project’s root—the
cargo.toml
file. Run a thorough check for any direct or indirect dependencies on Borsh and ensure they are on compatible terms.[dependencies] anchor-lang = "0.28.0" anchor-spl = "0.28.0" solana-program = "1.14.19" mpl-token-metadata = "1.12.0"
- Clean and update the Cargo environment: Next, clear the path by running
anchor clean
to get rid of any build artifacts causing obstacles. Then, refresh the environment by executingcargo update
. This helps update the dependencies as specified incargo.toml
. - Manage dependencies explicitly: If the trouble persists, it’s time to don the hat of a debugger. Start considering manually adjusting the versions of conflicting crates, which might require pinning certain dependencies to specific versions known for their compatibility.
cargo update -p solana-zk-token-sdk --precise 1.14.19 cargo update -p [email protected] --precise 0.9.3
These commands compelcargo
to use specific versions that could resolve conflicts. - Compile and test: Now that the changes are in place, it’s time to see if the problem is solved. Compile your updated project using
anchor build
. It’s always a best practice to run your tests too, ensuring no other parts of your program are affected by these changes.
Advanced tips to resolve Borsh serialization errors in Solana
Now that you know how to resolve the Borsh serialization error, let’s take a small detour to arm you with more knowledge for smoother expedition in the Solana development landscape.
- Understanding Borsh: Learning how Borsh serialization functions can be an enormous asset. A thorough understanding of how it serializes and deserializes data will enable you to debug and resolve serialization-related errors more effectively.
- Community and documentation: Don’t forget the power of community and documentation. Engage with the Solana and Rust communities for shared experiences, as there are likely many developers who encountered similar issues and can provide prompt solutions or workarounds. Additionally, the official documentation can be your best friend—it’s always a great place to refer to when you’re using certain dependencies.
What are blockstore errors when using Solana test validators?
While working with the Solana test validator, one common issue you might encounter is the blockstore error
. Easy to recognize but sometimes challenging to understand, it often arises due to the compatibility nuances between macOS and Solana’s required configurations.
In the pursuit of compatibility, Solana has integrated a set of tools and dependencies that ensure its smooth operation. However, occasionally this integration presents challenges, particularly for macOS users. The manifestation of this challenge is often a blockstore error
, an indication that something is not running harmoniously.
Why does this happen? The macOS operating system uses BSD tar by default, a variant that differs from GNU tar which Solana is compatible with. These differences in tar’s version lead to the blockstore error
.
Now that we’ve outlined the problem, let’s explore how to resolve it.
How to resolve blockstore errors in Solana test validators?
When encountering a blockstore error
, fear not. We have a step-by-step guide to lead you from confusion to clarity and from problem to resolution.
Step 1: Install Homebrew
To begin the process, we must first install Homebrew, a package manager making software package management on macOS easy. If you haven’t installed it yet, merely open your terminal and execute the following commands. It will download and install Homebrew on your device:
/bin/bash -c "$(curl -fsSL <https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh>)"
The system will guide you through the on-screen instructions to complete the installation. Next, let’s install GNU tar.
Step 2: Install GNU tar
With Homebrew at your disposal, installing GNU tar becomes a seamless task. Open your terminal and enter the command below:
brew install gnu-tar
This command will install GNU tar and incorporate it into a specific directory under Homebrew’s management. You’re halfway there! Now, we will take care of the PATH environment variable.
Step 3: Update the PATH environment variable
Updating your PATH environment variable is a vital step in resolving the blockstore error
. It allows you to set the preferences so that the terminal uses the newly installed GNU version of tar instead of its default BSD variant. Run the following command to update your PATH:
echo 'export PATH="/opt/homebrew/opt/gnu-tar/libexec/gnubin:$PATH"' >> ~/.zshrc
source ~/.zshrc
In running this command, you’re adding the directory containing GNU tar to the front of your sequencing PATH, ensuring that it’s the default command the terminal implements when invoking tar
. Now, it’s time to verify that we have successfully replaced BSD tar with GNU tar.
Step 4: Verify the installation
You can always ascertain whether your installation was successful by running the command:
tar --version
If the output correlates to GNU tar, congratulations! You have successfully replaced BSD tar with GNU tar, effectively preparing your device for an error-free Solana test validator experience. Now you just have to restart the validator.
Step 5: Restart the Solana test validator
With GNU tar now installed and designated as the default, you can confidently restart the Solana test validator. To start the validator without encountering the blockstore error
, type:
solana-test-validator
There you have it! Now your validator should run smoothly without any hindrances.
What is the Module parse failed
build error?
When building React Native projects that utilize the @solana
packages, you can often encounter a specific error. This error typically looks something like this:
Module parse failed: Identifier directly after number (3673:30)
You may need an appropriate loader to handle this file type.
| key: "isActive",
| value: function isActive() {
> var U64_MAX = Math.pow(2n, 64n) - 1n;
| return this.state.deactivationSlot === U64_MAX;
| }
This error message indicates a syntax or parsing issue. It’s usually triggered when the project’s build system doesn’t properly handle modern JavaScript features such as BigInt
literals. Understanding this error is the first step towards resolving it.
How to diagnose Module parse failed
build error?
To effectively resolve the error, it’s important to diagnose the problem and understand its root cause. Here are two key aspects to consider:
- BigInt and Math.pow(): The core of the error lies in the use of
Math.pow()
with BigInt (2n
,64n
).Math.pow()
is designed for numbers only and cannot handle BigInt. In JavaScript,2n ** 64n
would correctly use BigInt. However, certain transformations in the build process may convert this into a format usingMath.pow()
, which then leads to the process failing. - Compiler configuration: The JavaScript version targeted by the compiler is another aspect of this issue. The use of BigInt literals directly (
2n
) is supported in ES2020 and later. If the project’s compiler configuration targets an earlier JavaScript version, this can also trigger build errors.
By diagnosing the problem, you can better understand the error and find effective solutions to resolve it.
How to resolve the Module parse failed
build error?
And speaking of solutions, once the problem has been diagnosed, you can take steps to resolve the error. For example:
- Adjust compiler settings:
- Upgrade ECMAScript target: One of the simplest approaches is to ensure your build process, such as Babel, targets at least ES2020. You can set this in your Babel configuration (
babel.config.js
or.babelrc
) by specifying the target version:
{ "presets": [ ["@babel/preset-env", { "targets": ">= ES2020" }] ] }
- Custom Babel plugin configuration: If using specific plugins like
babel/plugin-transform-exponentiation-operator
, ensure they are correctly configured or updated to avoid transforming BigInt operations incorrectly.
- Upgrade ECMAScript target: One of the simplest approaches is to ensure your build process, such as Babel, targets at least ES2020. You can set this in your Babel configuration (
- Modify code directly: If you have control over the source code generating the error, consider replacing
Math.pow(2n, 64n) - 1n
with2n ** 64n - 1n
directly in the source or usingBigInt
functions explicitly, e.g.,BigInt(2) ** BigInt(64) - BigInt(1)
. - Report and collaborate: If modifying the library directly isn’t feasible, consider filing an issue on the library’s GitHub page (e.g.,
@solana-labs/solana
). This could prompt a permanent fix in the library itself that would benefit all users. - Test and verify: After making these changes, ensure to thoroughly test your application to verify that the build error is resolved and that it behaves as expected with Solana functionalities.
By implementing these solutions, you can effectively resolve the build error and move forward with your Solana development process.
What are Rust compilation errors in Solana?
Let’s start by understanding the build_hasher_simple_hash_one
compilation error that you as a Solana developer might encounter. It’s important to first know that this issue is generally due to a discrepancy between the version requirements of certain dependencies and the forked Rust compiler version adopted by Solana.
This inconsistency comes to light when you’re compiling a basic Solana program. An error message echoing the following lines may arrive on your screen:
error[E0658]: use of unstable library feature 'build_hasher_simple_hash_one'
--> src/random_state.rs:463:5
...
= note: see issue #86161 <https://github.com/rust-lang/rust/issues/86161> for more information
= help: add `#![feature(build_hasher_simple_hash_one)]` to the crate attributes to enable
The reason behind this error occurrence is related to the ahash
library, a dependency in your Solana project. Essentially, this library is trying to gain access to an unstable feature (build_hasher_simple_hash_one
) that was only perfected and stabilized in Rust version 1.71. However, Solana’s specific version of the Rust compiler might be seated a few steps below this Rust release.
Now that you’ve gained an adequate view of the issue, the following sections will guide you through a bread crumb trail of step-by-step solutions.
How to resolve Rust compilation errors in Solana?
Emerging victorious from this compilation error involves utilizing a few strategies. Here are the steps you can take:
Step 1: Check Rust and Solana versions
Your first order of action should be to become acquainted with the versions you’re employing in your project. This verification can be achieved by executing these commands in your terminal:
solana --version
rustup --version
rustup toolchain list
cargo build-sbf --version
These commands will show you the current versions of solana-cli
, rustup
, and the specific rustc
version employed by Solana.
Step 2: Pin the ahash
version
Solving this issue may involve anchoring the ahash
library to a version that the older Rust compiler version, employed by Solana, can comprehend. You can achieve this by indicating the ahash
version in your cargo.toml
file:
[dependencies]
ahash = "=0.8.6"
Alternatively, you can update ahash
to a specific version using the following command:
cargo update -p [email protected] --precise 0.8.6
Step 3: Use Rust features
If fiddling with the ahash
version seems troublesome, another manoeuver would involve utilizing the Rust feature flag. This can help enable the unstable feature within your development environment. Insert the following line to the peak of your main library file (lib.rs
or main.rs
):
#![feature(build_hasher_simple_hash_one)]
Bear in mind, this method is like a makeshift bridge. It provides a temporary fix and should be employed with caution as it hinges on unstable Rust features.
Step 4: Monitor Solana updates
Since Solana operates with a distinct fork of Rust (solana-cargo-build-sbf
), it’s crucial to keep track of modifications to Solana’s Rust version. Keenly monitor Solana changes pertinent to their Rust version through the Solana GitHub issue tracker. Check in on issues related to this matter, like #33504.
Step 5: Use an alternative dependency
If ahash
continues to play the villain and your project allows for a little wiggle room, think about switching over to a different hashing library. This new library should either be harmonious with older versions of Rust or not bank heavily on unstable features.
Rust compilation error best practices for Solana developers
Now that we’ve walked you through the problem and its possible solutions, here are some best practices to prevent similar hitches or to handle them efficiently if they occur in the future.
Version management
Timely updates and effective management of toolchain versions with rustup
and solana-cli
are crucial to maintaining the smooth running of Solana development. It’s not just about installing these tools, but more so about making sure they’re kept up-to-date and that they maintain compatibility with each other.
Dependency audit
Sometimes, indirect updates from your dependencies may catch you off-guard. Make it a habit to periodically review and audit your dependencies. This practice will help you keep acquaintances with the versions you’re using and enable you to preempt any potential issues.
Community engagement
Become a participant, not just a spectator, in the Solana development community. Platforms like Chainstack, StackExchange, GitHub, or Solana’s official forums, not only serve as a knowledge base but also provide tried-and-tested troubleshooting advice from experienced peers and experts in the field. Staying active in these communities can offer unexpected insight and potential solutions to issues you might face in the future.
With these practices in place combined with a deeper understanding of Rust’s version compatibility, you’ll be on your path to becoming a seasoned Solana developer. You’ll find that issues like the build_hasher_simple_hash_one
actually serve as stepping stones, building your skills in the vibrant landscape of Solana software development.
Further reading
Expand your Solana knowledge and skills with these comprehensive Chainstack resources:
- The ultimate Solana developer guide: Master Solana development all across the board with this ultimate guide, covering everything from pastable snippets to advanced integrations.
- Mastering Solana series: Explore Solana essentials like token swaps with Raydium SDK, SPL token transfers, account retrieval methods, and getTokenLargestAccounts RPC insights.
- Solana-web3.js Tutorial: Learn how to use the solana-web3.js method in just 7 minutes to mint an SPL token, transfer tokens, and delegate tokens.
- Querying and analyzing Solana data from Raydium with Python: Learn about Raydium, a leading DEX on Solana, and explore how DeFi enables token exchanges across chains and fiat.
- Powerful Chainstack infrastructure optimized for Solana: Run high-performing Solana RPC nodes and APIs in minutes on a platform built for scale with Chainstack.
- How to run a Solana RPC node: Learn how to run your Solana RPC node instance on Chainstack, connected to the mainnet beta and complete with metric monitoring.
- Solana tool suite: Learn how to interact with your Chainstack Solana node and develop DApps.
- Solana glossary: Get a better understanding of key Solana terminology and its definitions.
Bringing it all together
Troubleshooting common Solana development errors is essential for maintaining a smooth workflow and ensuring the stability of your DApps. By understanding and resolving issues such as Rust version conflicts, Borsh serialization errors, blockstore errors, and module parse errors, you can enhance your development process and build more reliable applications.
Remember to manage your dependencies, stay engaged with the community, and keep your toolchains up to date. With these practices, you’ll be well-equipped to navigate the complexities of Solana development and achieve success in your projects.
Power-boost your project on Chainstack
- Discover how you can save thousands in infra costs every month with our unbeatable pricing on the most complete Web3 development platform.
- Input your workload and see how affordable Chainstack is compared to other RPC providers.
- Connect to Ethereum, Solana, BNB Smart Chain, Polygon, Arbitrum, Base, Optimism, Avalanche, TON, Ronin, zkSync Era, Starknet, Scroll, Aptos, Fantom, Cronos, Gnosis Chain, Klaytn, Moonbeam, Celo, Aurora, Oasis Sapphire, Polygon zkEVM, Bitcoin and Harmony mainnet or testnets through an interface designed to help you get the job done.
- To learn more about Chainstack, visit our Developer Portal or join our Discord server and Telegram group.
- Are you in need of testnet tokens? Request some from our faucets. Multi-chain faucet, Sepolia faucet, Holesky faucet, BNB faucet, zkSync faucet, Scroll faucet.
Have you already explored what you can achieve with Chainstack? Get started for free today.