Solana-web3.js tutorial – Send a Solana transaction in 3 Minutes
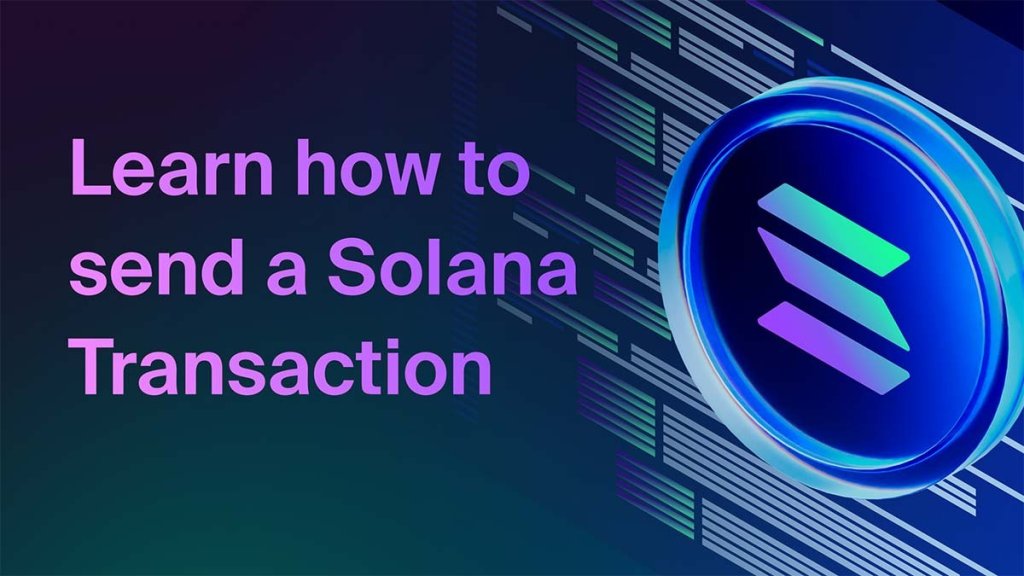
A few weeks ago, we published another article about how to mint an SPL token on Solana, which is a foundational component of Solana development. So, following this trend of covering foundational tutorials in order to support you as a Solana developer, in this tutorial you will learn how to send a Solana transaction using solana-web3.js.
Install solana-web3.js library
To get started you’ll first have to install the solana-web3.js library, with node package manager. You can do that with npm install.
npm install @solana/web3.js
We will also need to install a base58 library. You don’t have to do this if you plan on using an array type private key, but in this case, we plan on using a standard string private key base58, the type of private key you get from a Phantom wallet for example.
So we will need this base58 library in order to do some conversions.
npm install bs58
Define variables
Alright, so now that you have solana-web3.js and bs58 installed, we can go ahead and define your variables in the index file.
const web3 = require("@solana/web3.js");
const bs58 = require("bs58");
Connect to the Solana blockchain
Now you need to connect to the Solana blockchain itself through a connection variable.
Deploy your own Solana node with Chainstack for free
If you do not have a Chainstack account yet, you can sign up for free in order to follow along with this tutorial.
After you have your account, you can go ahead and deploy a node by following the steps in the video below.
Once your node is running, you can click on it to see its details.
Scroll to the bottom of the page and grab the HTTPS endpoint.
Define the connection variable.
const connection = new web3.Connection("copy paste your https endpoint here")
Great, now we can move on to creating the Solana account that is going to send this transaction.
Define the private key variable
const privateKey = new Uint8Array(bs58.decode(process.env['PRIVATE_KEY']));
The code above defines a new variable, called privateKey, and is setting it up to be of type Uint8Array, which calls a base58 object. The code then calls the decode method on the object.
Derive the account from the private key
Now we can go ahead and derive the account from the private key that we’ve just defined.
const account = web3.Keypair.fromSecretKey(privateKey);
Define the account that we are sending to
We will create a fresh account to send funds to.
const account2 = web3.Keypair.generate();
The second account has both a private, and a public key, but in order to send funds to the account, we only need the public key, which is what we will be using here.
Define & push the transaction
(async () => {
const transaction = new web3.Transaction().add(
web3.SystemProgram.transfer({
fromPubKey: account.publicKey,
toPubKey: account2.publicKey,
lamports: web3.LAMPORTS_PER_SOL * 0.001,
}),
);
const signature = await web3.sendAndConfirmTransaction(
connection,
transaction,
[account],
);
})();
We defined a transaction object and our signature, which will push the transaction on the Solana blockchain.
Recap of what you did in this tutorial
Imported the packages.
Defined a connection variable using a Chainstack node.
Defined your private key and derived an account from it.
Created a 2nd account to send the Solana to.
Created a transaction object using from, to, and value.
Sent that transaction and stored it in a variable called signature.
Let’s put it to the test. Go over to the console and run the code.
Now, if you check your Phantom wallet, you should see the transaction in there.
If you are looking for another Solana tutorial, check out our solana-web3.js tutorial.
Power-boost your project on Chainstack
- Discover how you can save thousands in infra costs every month with our unbeatable pricing on the most complete Web3 development platform.
- Input your workload and see how affordable Chainstack is compared to other RPC providers.
- Connect to Ethereum, Solana, BNB Smart Chain, Polygon, Arbitrum, Base, Optimism, Avalanche, TON, Ronin, zkSync Era, Starknet, Scroll, Aptos, Fantom, Cronos, Gnosis Chain, Klaytn, Moonbeam, Celo, Aurora, Oasis Sapphire, Polygon zkEVM, Bitcoin and Harmony mainnet or testnets through an interface designed to help you get the job done.
- To learn more about Chainstack, visit our Developer Portal or join our Discord server and Telegram group.
- Are you in need of testnet tokens? Request some from our faucets. Multi-chain faucet, Sepolia faucet, Holesky faucet, BNB faucet, zkSync faucet, Scroll faucet.
Have you already explored what you can achieve with Chainstack? Get started for free today.